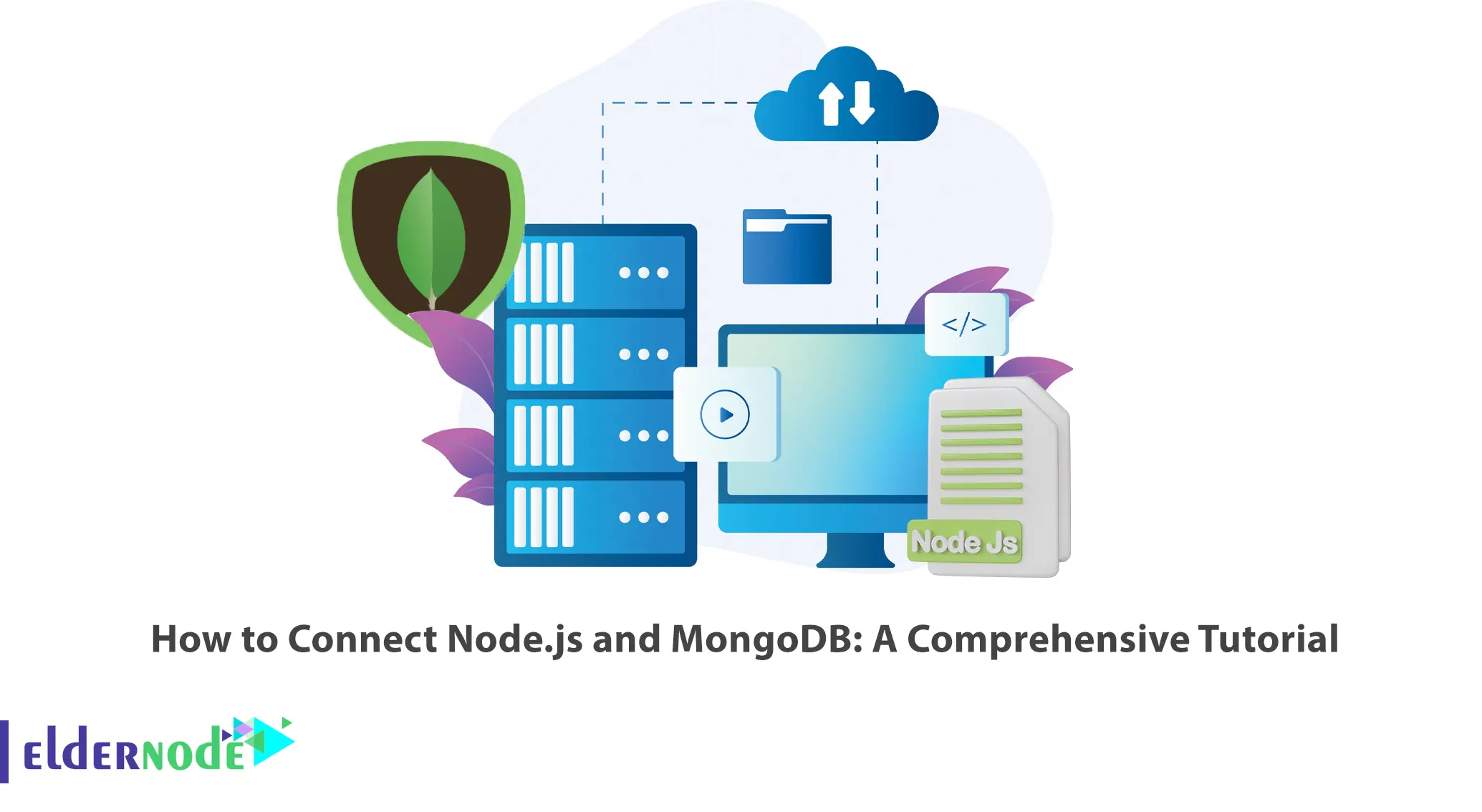
MongoDB database has always been popular with Node.js developers due to the type of storage and queries it has. In this article, we intend to fully teach you How to Connect Node.js and MongoDB. If you want to buy VPS server, you can visit the various plans offered on the Eldernode website.
Table of Contents
Tutorial Connect Node.js and MongoDB
What is Node.js?
Node.js is a server-side platform based on the Google Chrome JavaScript engine (V8 Engine). Node.JS provides everything you need to run a program written in JavaScript.
Mr. Ryan Dahl introduced Node.JS in 2009 to show that JavaScript is more powerful than just being used for front-end dynamic web pages. In fact, with the help of Node.js, the JavaScript programming language is executing in the server environment instead of in the browser. Node.js allows you to easily and simply write scalable and large networked applications.
JavaScript has been evolving since 1995. Although this language did not have a successful presence on the server side until a long time ago, and the efforts made by the programmers faded from the minds of other developers over time. Until the introduction of Node.js in 2009, the tide turned and over time JavaScript was used more and more on the server side.
What are the uses of Node.js?
One of the environments that allows programmers to run their JavaScript code on the server side is Node.js. In the following, we will explain some important applications of Node.js to you.
1. Creating single page applications (SPA)
SPA refers to programs that all parts are implemented in one page. Since Node.js supports asynchronous programming well, it is considered a good choice for building SPA applications.
2. Making RTA programs
RTA stands for real-time app. That is, programs that have various changes in real time. In general, interactive applications, project management tools, video and audio conferencing, and other RTA applications perform heavy I/O operations.
3. Creating a chat room
One of the most famous examples of RTA applications are chat rooms and messengers. In fact, Node.js is a great choice for building a chat room. If you intend to make such a product, you should pay attention to various things such as tolerating a lot of user traffic, the lightness of the product, the high speed of sending messages, etc. All these things can implement on the server side with the help of Node.js and a JavaScript framework like Express.js.
4. Creating online games under web browser
With the help of Node.js, you can develop web games. In fact, by combining HTML5 technologies and JavaScript tools, you can create attractive 2D games such as Ancient Beast or PaintWar.
What is MongoDB?
MongoDB is one of the most famous No SQL databases. This database has a flexible structure and is mostly using in projects with a large amount of data. This database is a free and open source platform and works with Document-Oriented data model and can be used on Windows, Macintosh and Linux. Data values storing in MongoDB are using with two primary keys (Primary Key) and secondary key (Secondary Key).
MongoDB contains an array of values. These values are in the form of documents that contain different types of data with different sizes. This issue has made MongoDB able to store data with a complex structure such as hierarchical or array data.
Features of MongoDB
Due to the document-oriented data storage model, MongoDB is much more flexible and scalable compared to relational databases, and it solves many business needs.
This database uses sharding to divide data and manage the system better. Sharding means breaking into pieces and is done at the top load of the network. In such a way that the database is dividing into several subsections to make the process of responding to the requests that come from the server easier.
Data can be accessed with two primary and secondary keys, and each field can be keyed. This makes data access and processing time very fast.
Replication is another important feature of MongoDB. In this technique, copies are made of a data as the main data and other parts of the database system are storing. If this data is lost or corrupted, the copied data will use as the original and alternative data.
In the rest of this article, after learning how to install MongoDB Node.js Driver, we will teach you how to connect Node.js and MongoDB.
How to Install the MongoDB Node.js Driver
In this section, we are going to teach you how to install MongoDB Node.js Driver. The reason for installing the MongoDB Node.js driver is to allow you to easily interact with MongoDB databases from within Node.js applications. Therefore, you need a driver to connect to the database and perform the activities you want.
You can easily install MongoDB Node.js by running the following command:
npm install mongodb
After you have installed the MongoDB Node.js Driver, you should now create a free MongoDB Atlas cluster.
Considering that you need a MongoDB database, we suggest you use Atlas. It should note that this database is fully managing as a service.
1. To do this, just go to Atlas and create a new cluster in the free row.
2. Then you can download the sample data.
3. The last step is to prepare your cluster for connection.
4. Establish a cluster connection, you need to go to your cluster in Atlas and click CONNECT.
5. Then the Cluster Connection Wizard will be shown to you.
Note that the Wizard will ask you to add the current IP address to the IP access list. To add an IP, you need to create a MongoDB user.
6. Then you need to choose a connection method.
7. In the next step, you can choose Connect Your Application.
Note: You must select Node.js and version 3.6 or higher.
8. Finally, you can copy the provided connection string.
How to Connect to Database from a Node.js
Now you can learn about how to connect Node.js and MongoDB. We are going to write a Node.js script that connects to the database. Doing so will list the databases in the cluster. The first step is to import MongoClient. You can use an instance of MongoClient using the following command. By doing this you can use to connect to a cluster, access the database in that cluster and close the connection to that cluster:
const {MongoClient} = require('mongodb');
We recommend that you create an asynchronous function called main() in the next step. Using this function that you see, you will be able to connect to your MongoDB cluster and call the required functions:
async function main() { // we'll add code here soon }
You must create a constant for your connection URI inside main(). When you paste the connection string, update <username> and <password> as the user credentials you created in the previous section.
Connection string contains a <dbname> placeholder. In the following commands, considering that the sample_airbnb database is used, it is necessary to replace <dbname> with sample_airbnb:
/** * Connection URI. Update <username>, <password>, and <your-cluster-url> to reflect your cluster. * See https://docs.mongodb.com/ecosystem/drivers/node/ for more details */ const uri = "mongodb+srv://<username>:<password>@<your-cluster-url>/test?retryWrites=true&w=majority";
Create an instance of MongoClient:
const client = new MongoClient(uri);
It is now possible to connect to the cluster with MongoClient. client.connect() returns a promise. Calling client.connect() with await means that further execution should blocked until that operation completes:
await client.connect();
In the next step, create a function to print the names of the databases in this cluster. Therefore, you can call a function called listDatabases() using the following command:
await list Databases(client);
To handle errors, close calls to functions that interact with the database by executing the following command:
try { await client.connect(); await listDatabases(client); } catch (e) { console.error(e); }
Run the following command to make sure the clusters are closed:
finally { await client.close(); }
Then, you can send the errors to the console:
main().catch(console.error);
Finally, by putting together the main() function and the calling commands, it will be like the following commands:
async function main(){ /** * Connection URI. Update <username>, <password>, and <your-cluster-url> to reflect your cluster. * See https://docs.mongodb.com/ecosystem/drivers/node/ for more details */ const uri = "mongodb+srv://<username>:<password>@<your-cluster-url>/test?retryWrites=true&w=majority"; const client = new MongoClient(uri); try { // Connect to the MongoDB cluster await client.connect(); // Make the appropriate DB calls await listDatabases(client); } catch (e) { console.error(e); } finally { await client.close(); } } main().catch(console.error);
You can list the databases in the cluster and print and view the results in the output:
async function listDatabases(client){ databasesList = await client.db().admin().listDatabases(); console.log("Databases:"); databasesList.databases.forEach(db => console.log(` - ${db.name}`)); };
Save the file to apply the changes and name it something like connect.js.
How to Execute Node.js Script
After you have successfully completed the mentioned steps, now it is time to test the code. So you can easily run your script with the help of the following command:
node connection.js
Output:
Databases:
– sample_airbnb
– sample_geospatial
– sample_mflix
– sample_supplies
– sample_training
– sample_weatherdata
– admin
– local
Conclusion
In this post, we tried to introduce Node.js and MongoDB to you. Then we taught you How to Connect Node.js and MongoDB. If you have any questions, you can ask the support experts in the comments section.